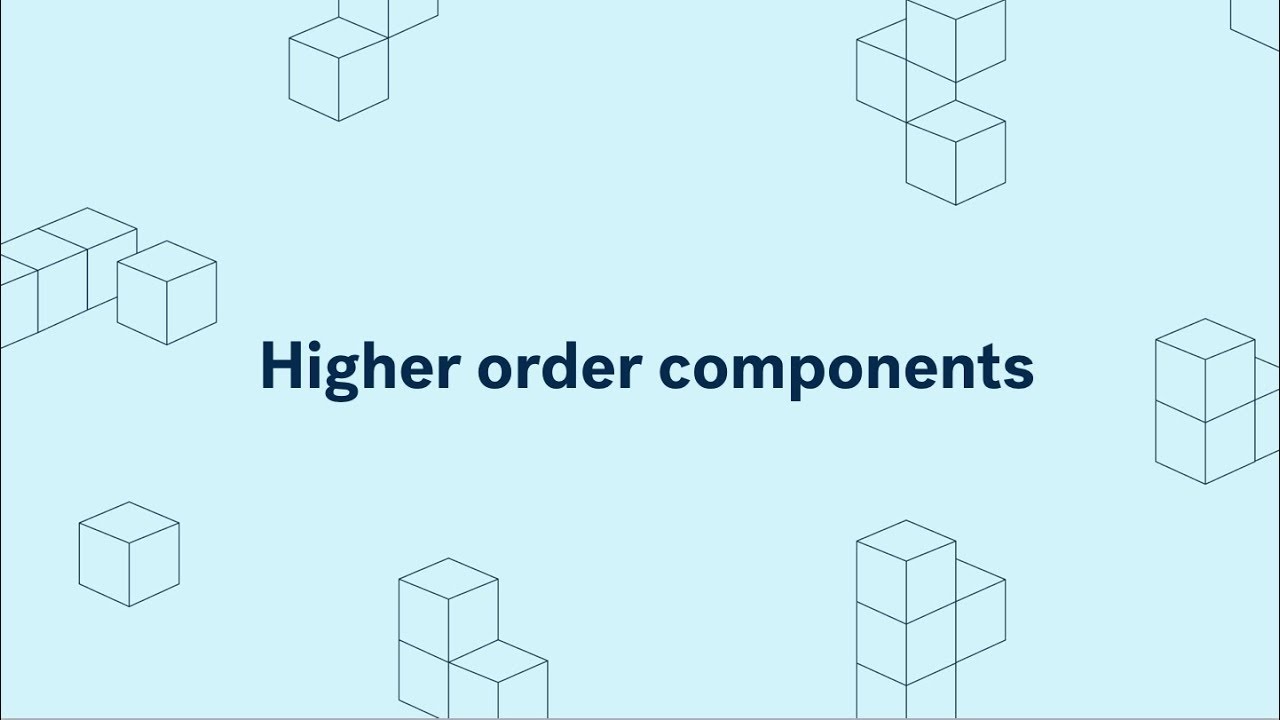
TUTORIAL Higher order components in React YouTube
The key idea to keep in mind when exploring higher-order components is they're intended to support code reuse between components. That's it. That's why they exist. Higher-order components are so called because they're components that consume and return another component. The name higher-order components is a reference to higher-order.

A Gentle Introduction to HigherOrder Components in React Envato Tuts+
Higher-Order Component is an advanced function, which reuses component logic in a React Component and returns an enhanced new component. It wraps commonly used processes to allow their use by different components, such as state modification or props change. Components are wrapped in HOCs, whose goal is to improve them without changing their original constructions.

ReactJS HigherOrder Components
Higher-Order Components in React, also known as HOCs, are an advanced component pattern in React (next to Render Props Components ). Higher-Order Components can be used for multiple use cases. I want to pick out one use case, the conditional rendering with Higher-Order Components, to give you two outcomes from this article as a learner.

HigherOrder Components in React Aleksandr Hovhannisyan
ReactJS Higher-Order Components, or HOCs, are advanced techniques for reusing component logic. Think of it as a JavaScript function. Simply put, it is a pattern that is derived from React's compositional nature. The subsequent sections will shed more light on their method of operation, when to use them, and potential use cases.
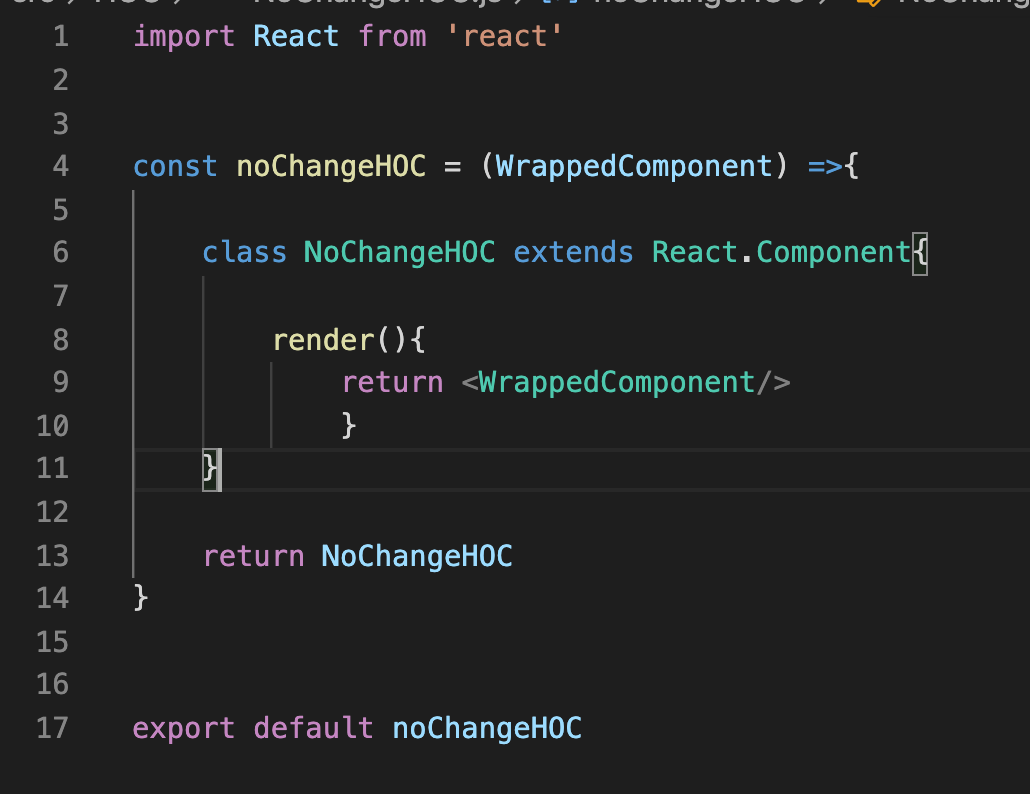
HigherOrder Components in React. A higherorder component (HOC) is a
With that in mind, we want our higher-order component (which we'll call withHover) to be able to encapsulate that hover logic in itself and then pass the hovering state to the component that it renders. That will allow us to prevent duplicating all the hover logic and instead, put it into a single location (withHover).Ultimately, here's the end goal.
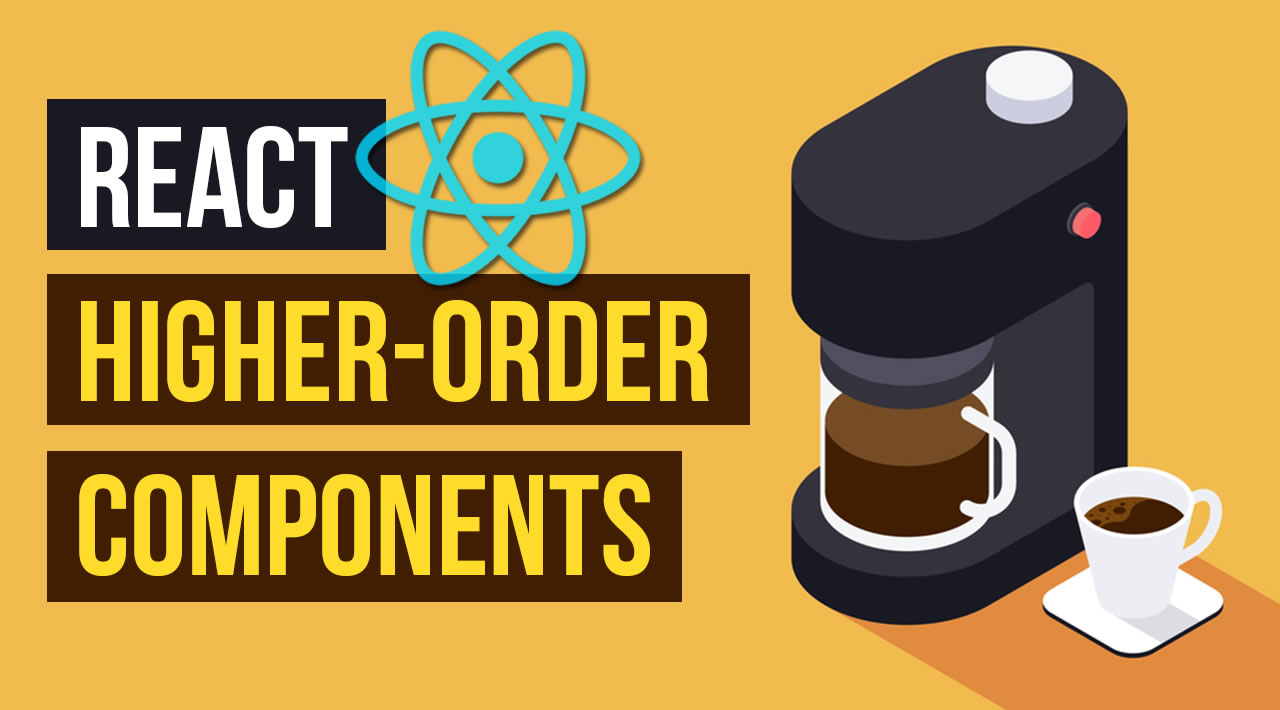
The Complete Guide to HigherOrder Components in React
Higher-order components (HOCs) are a powerful feature of the React library. They allow you to reuse component logic across multiple components. In React, a higher-order component is a function that takes a component as an argument and returns a new component that wraps the original component. HOCs allow you

What is the Advantage of HigherOrder Components in React.JS
The React docs say that higher-order components take a component and return a component. The use of higher-order components comes in handy when you are architecturally ready for separating container components from presentation components. The presentation component is often a stateless functional component that takes props and renders UI.
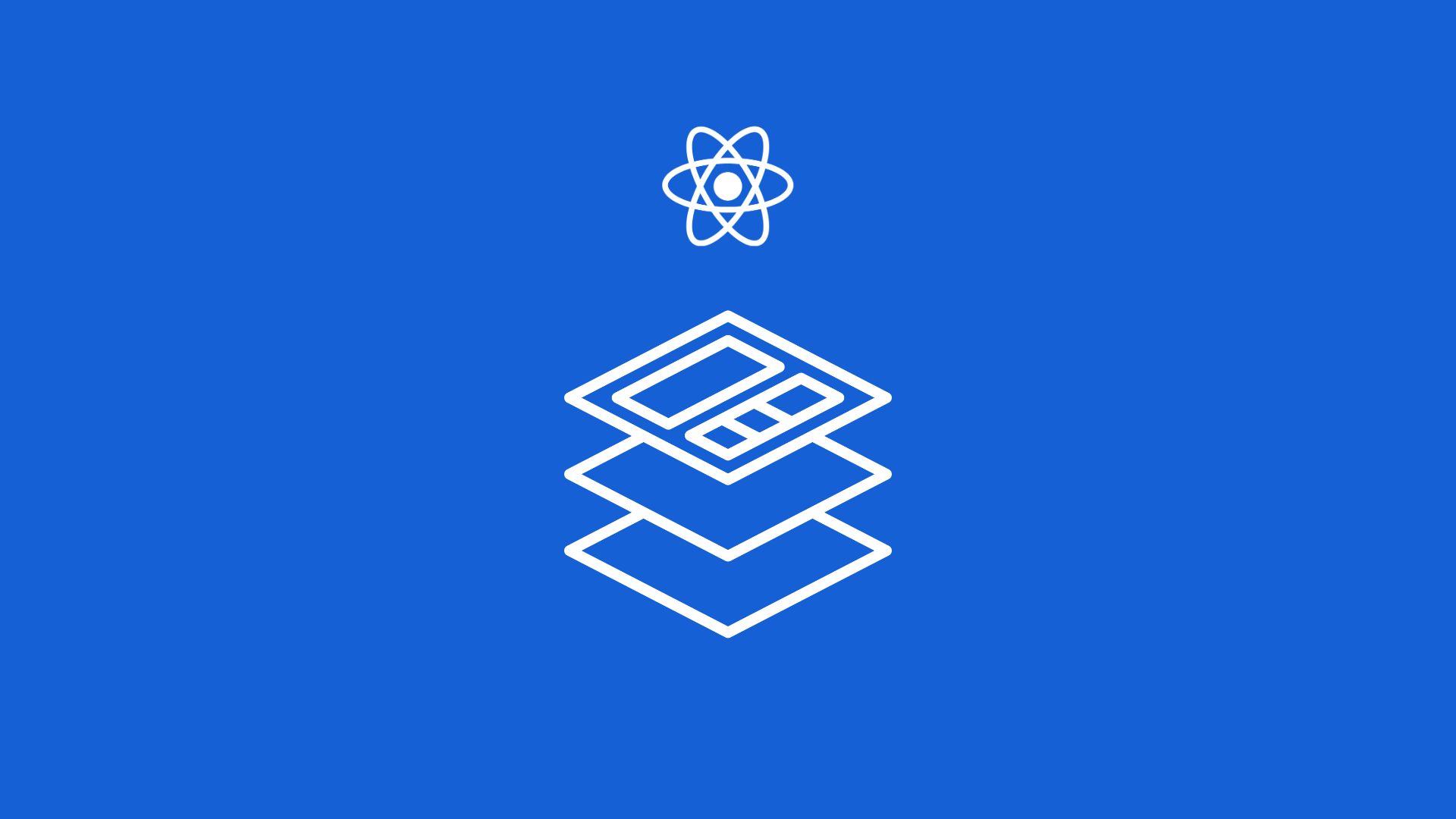
What is higher order component in React? Explanation with example
At the most basic level, React Higher-Order Component is the function that returns a class and is the implementation of functional programming principles in your codebase. It is also possible to pass higher order components into other higher order components since typically they take components as input and return other components as output.
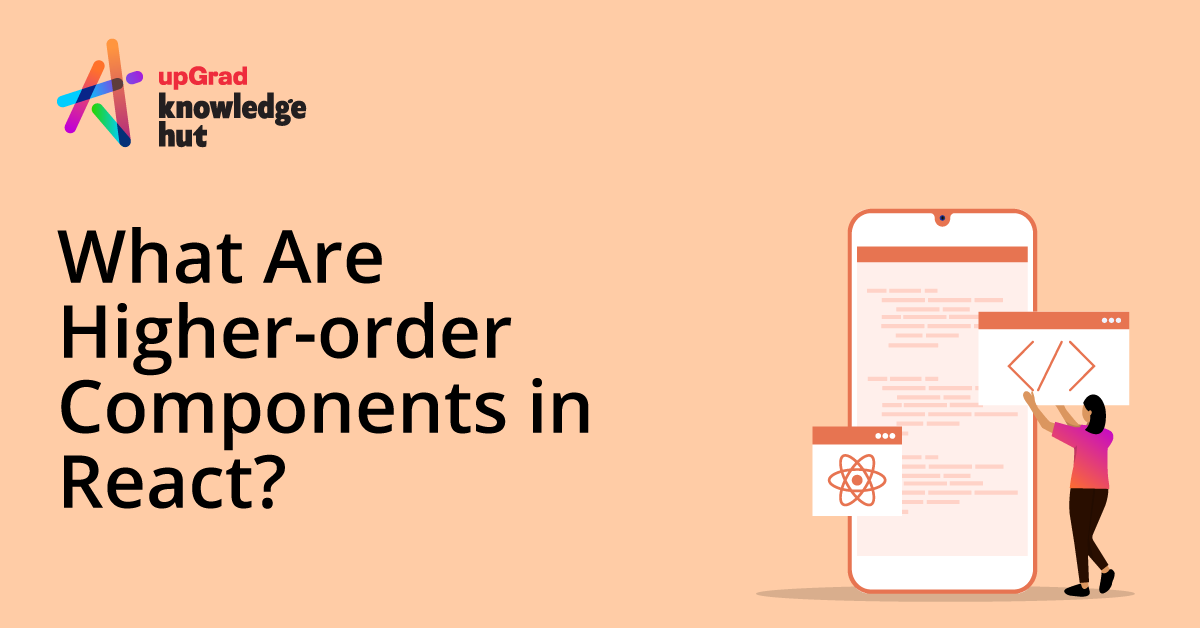
What Are Higherorder Components in React?
Above, we create a higher-order functional component named withName (). Then, we return an anonymous class component inside that renders the component wrapped in the HOC. And we assign a value to the prop of the wrapped component. const NewComponent = withName(Hello); Here, we create a new component named NewComponent.
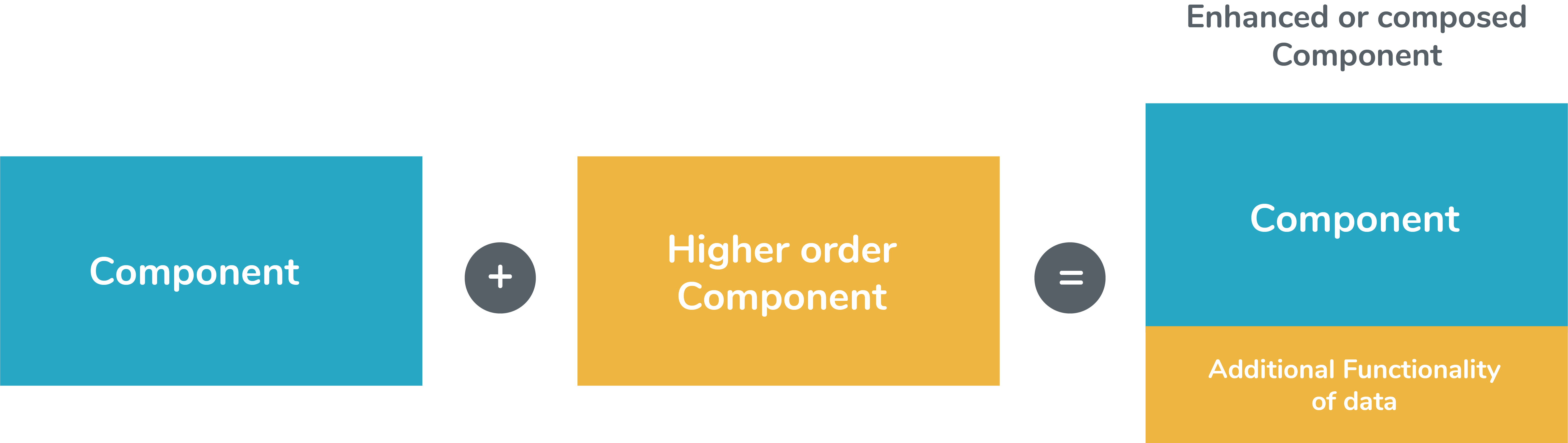
What are Higher Order Components in Reactjs?
Introducing higher-order components. Let's now talk about the nature of the higher-order function withFilterProps. In React's vocabulary, such a function is called a higher-order component (HoC). Just as the higher-order function creates a new function, the HoC creates a new component.
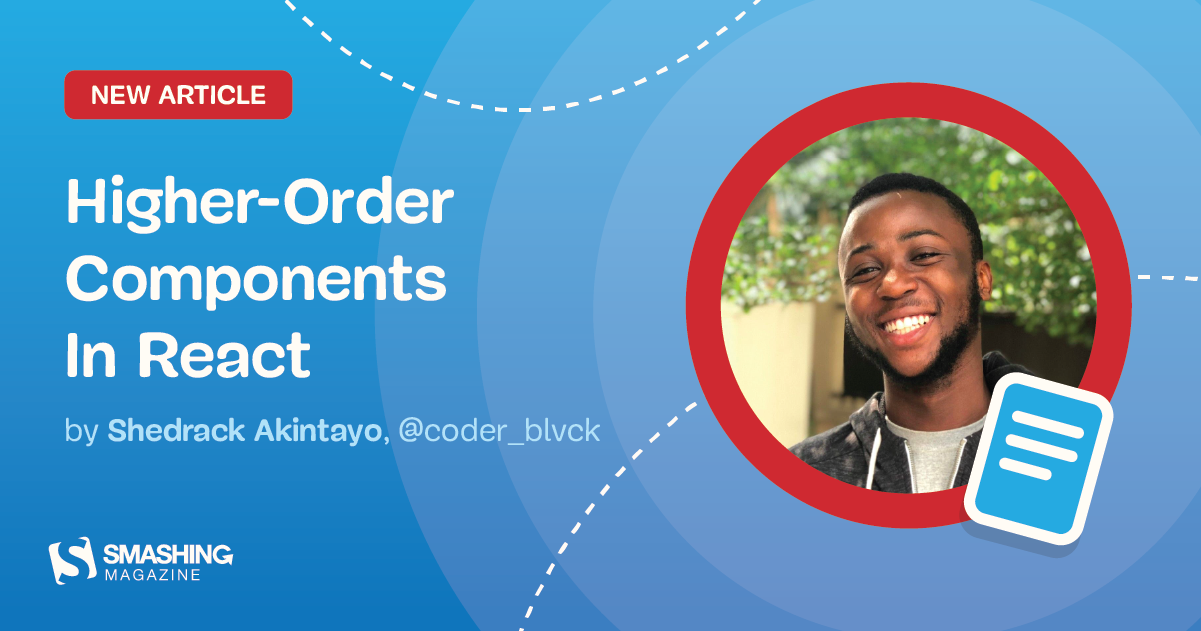
HigherOrder Components In React
Before the introduction of hooks, higher-order components were widely used for accessing context and any external data subscriptions. Redux connect or react-router's withRouter functions are higher-order components: they accept a component, inject some props into it, and return it back.

React 18 Higher Order Component Function Component Tutorial PositronX.io
Higher Order Components is a great Pattern that has proven to be very valuable for several React libraries. In this Post we will review in detail what HOCs are, what you can do with them and their.
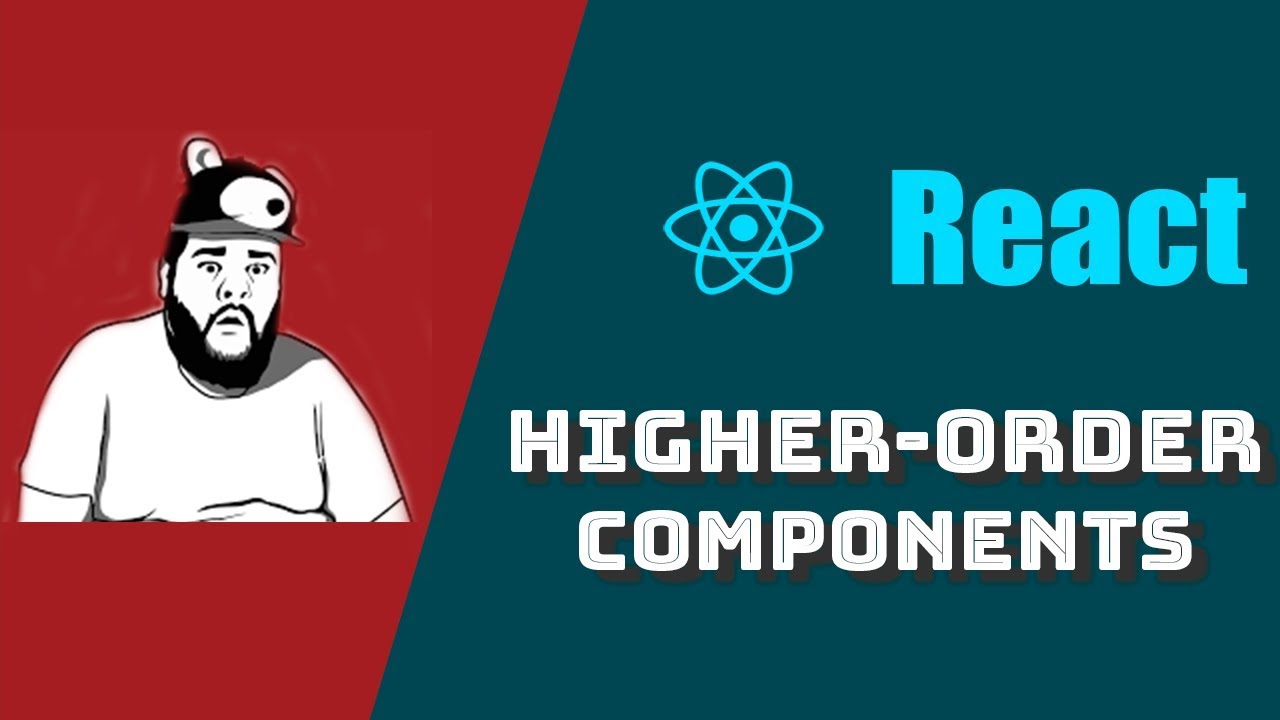
React Higher Order Components Tutorial YouTube
Higher-order components are not commonly used in modern React code. A higher-order component (HOC) is an advanced technique in React for reusing component logic. HOCs are not part of the React API, per se. They are a pattern that emerges from React's compositional nature. Concretely, a higher-order component is a function that takes a.
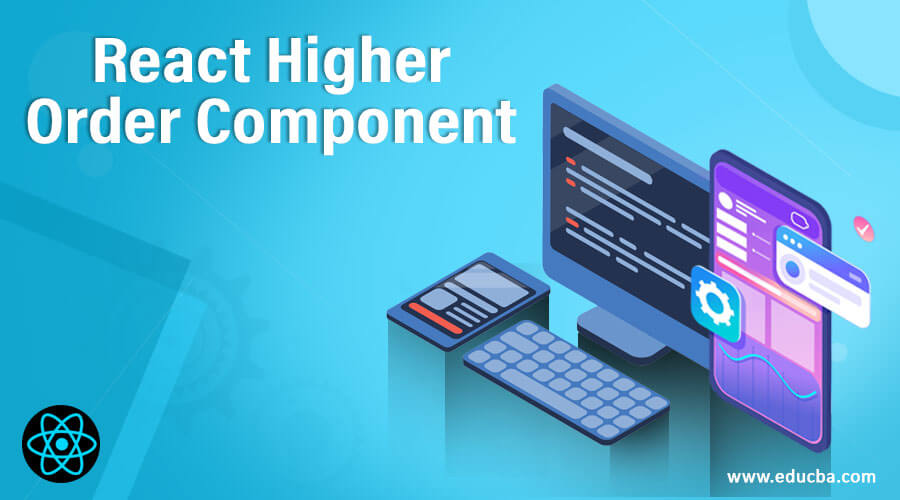
React Higher Order Component Examples with Code Implementation
A higher-order component is a function that takes in a component and returns a new component. Using code, we can rewrite the above statement like so: const newComponent = higherFunction(WrappedComponent); In this line: newComponent: Will be the enhanced component.
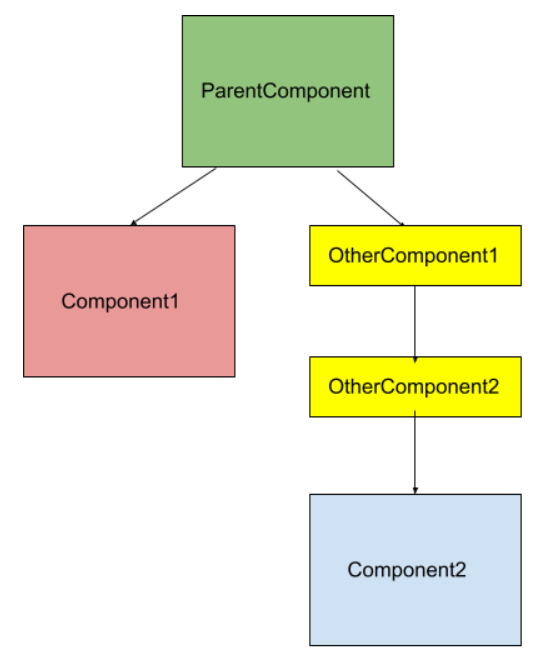
HigherOrder Components in React Coding Ninjas CodeStudio
Definition. Higher Order Component (HOC): an abstraction over a component. When given a component (and perhaps some other parameters), they return a new component. From the perspective of the JavaScript language: a higher-order component is a function that takes a component and returns a new component.

React HigherOrder Components Explained with Examples YouTube
In a nutshell, higher-order components are component factories that take a component, inject props into it, and return the modified component. As we saw, you can compose higher-order components and even combine them with React's Context API to write powerful, reusable code. Attributions. Social media preview: Photo by Patrick Hendry .