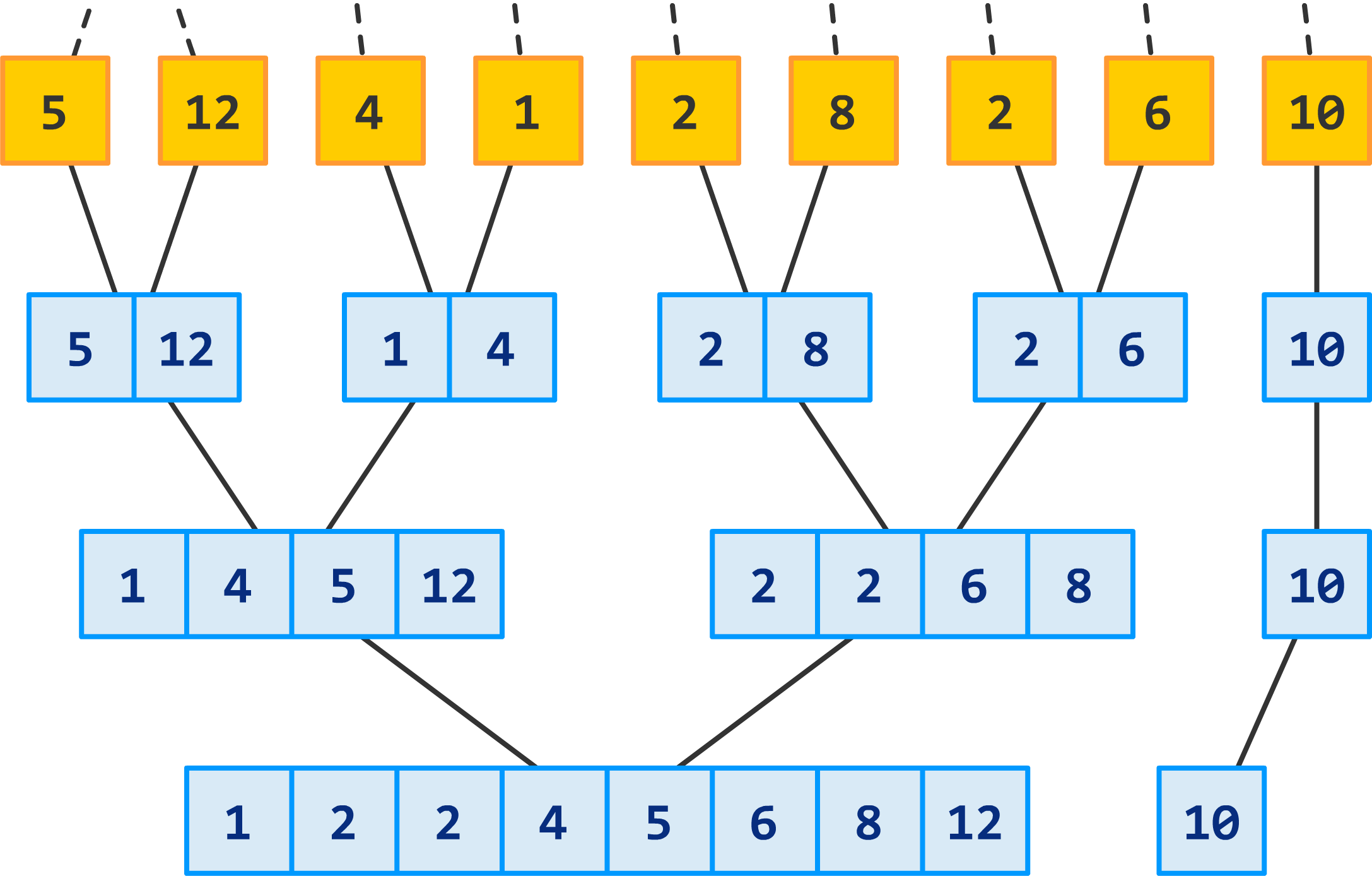
All About Mergesort
Like QuickSort, Merge Sort is a Divide and Conquer algorithm. It divides the input array into two halves, calls itself for the two halves, and then it merges the two sorted halves. The merge() function is used for merging two halves. The merge(arr, l, m, r) is a key process that assumes that arr[l..m] and arr[m+1..r] are sorted and merges the.

Merge Sort and its analysis
The way Merge Sort works is: An initial array is divided into two roughly equal parts. If the array has an odd number of elements, one of those "halves" is by one element larger than the other. The subarrays are divided over and over again into halves until you end up with arrays that have only one element each.

Learn Merge Sort in 13 minutes 🔪 YouTube
Merge sort is one of the most efficient sorting algorithms. It works on the principle of Divide and Conquer based on the idea of breaking down a list into several sub-lists until each sublist consists of a single element and merging those sublists in a manner that results into a sorted list.. Merge Sort Working Rule. The concept of Divide and Conquer involves three steps:
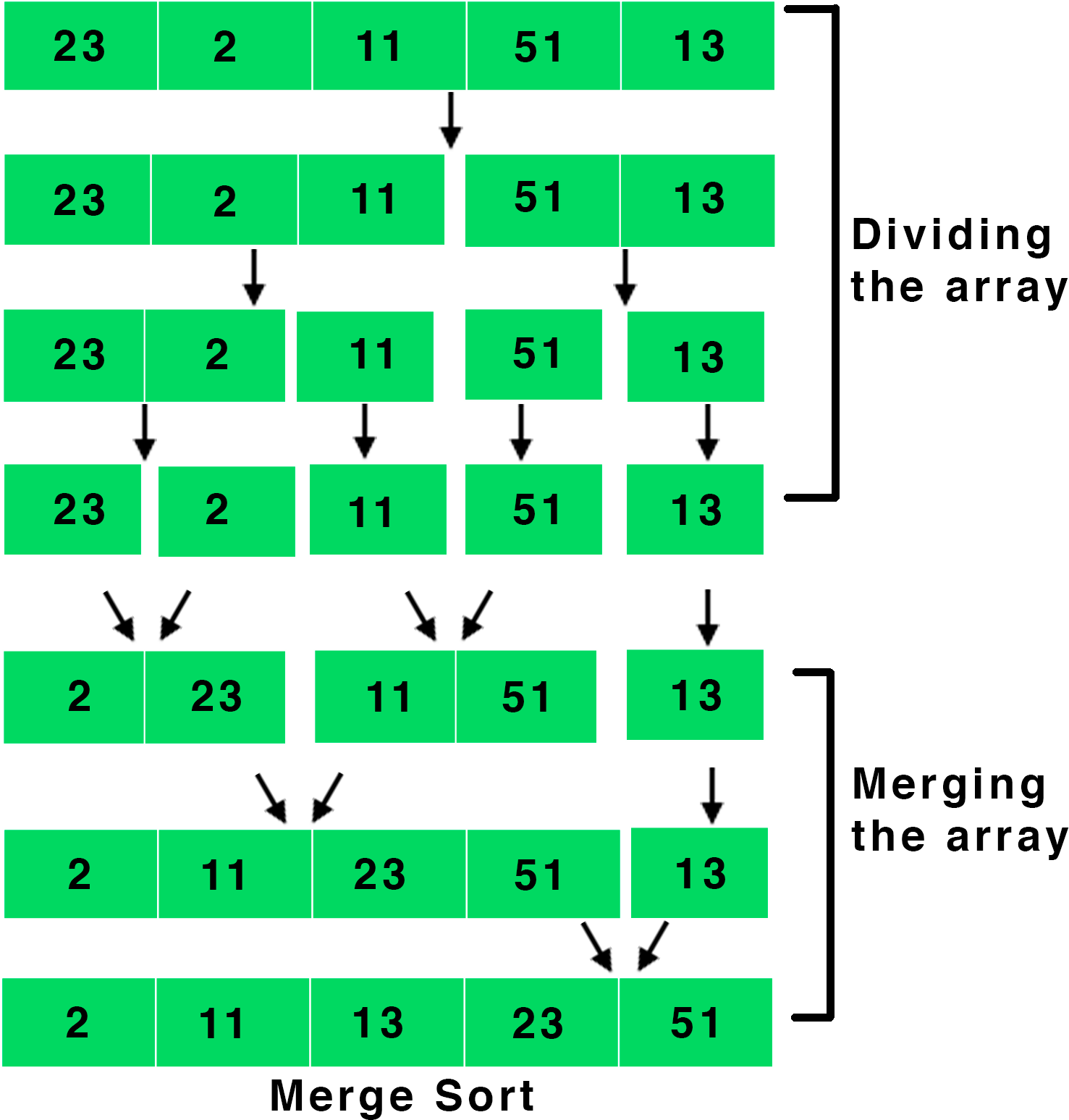
Merge Sort
return. q = (p+r)/2. mergeSort(A, p, q) mergeSort(A, q+1, r) merge(A, p, q, r) To sort an entire array, we need to call MergeSort (A, 0, length (A)-1). As shown in the image below, the merge sort algorithm recursively divides the array into halves until we reach the base case of array with 1 element. After that, the merge function picks up the.
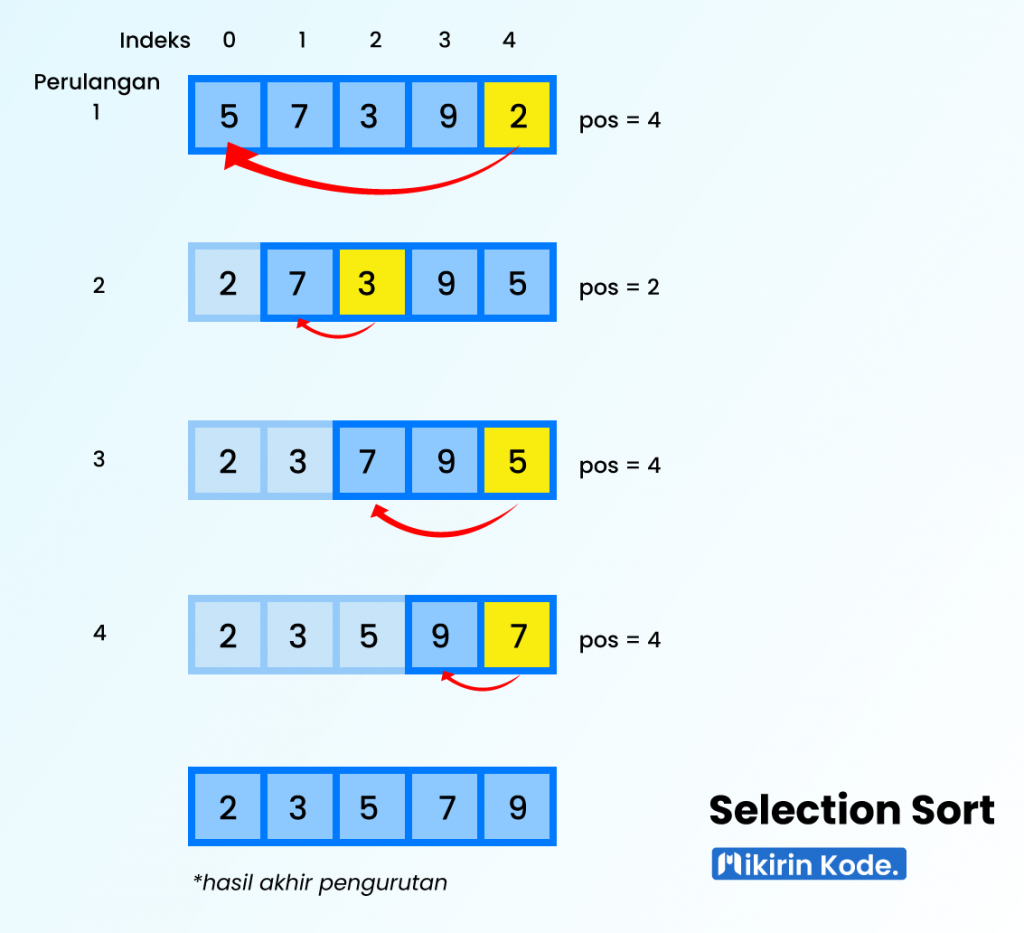
Selection Sort Algoritma Pengurutan MikirinKode
Pengertian Algoritma Merge Sort. Algoritma Merge Sort adalah salah satu metode pengurutan data yang berbasis perbandingan dan memanfaatkan teknik "divide and conquer" atau "bagi dan taklukkan". Metode ini efisien untuk mengurutkan kumpulan data dengan ukuran besar. Pada dasarnya, algoritma Merge Sort memecah daftar data menjadi bagian.
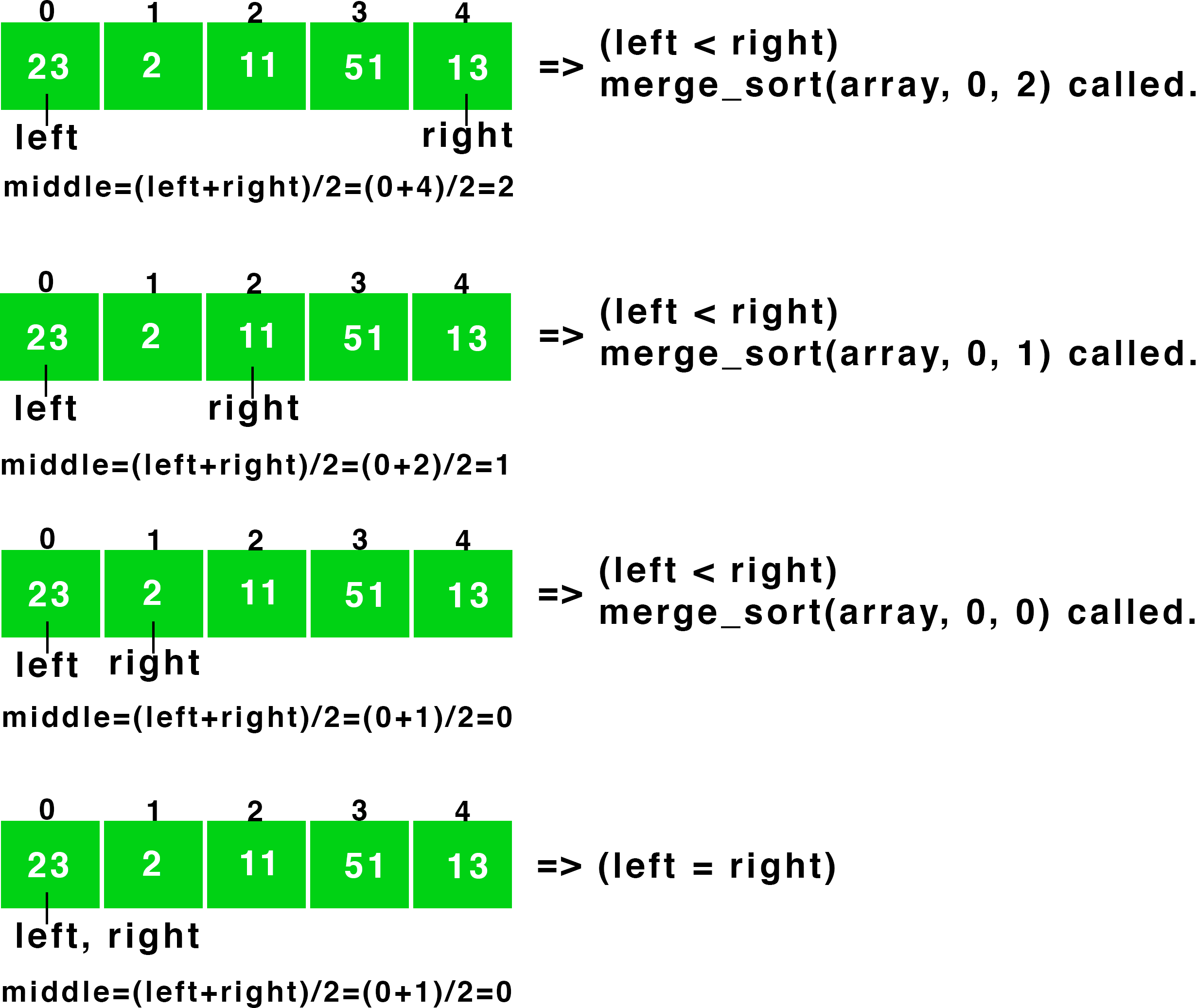
Merge Sort
Merge sort is defined as a sorting algorithm that works by dividing an array into smaller subarrays, sorting each subarray, and then merging the sorted subarrays back together to form the final sorted array.. In simple terms, we can say that the process of merge sort is to divide the array into two halves, sort each half, and then merge the sorted halves back together.
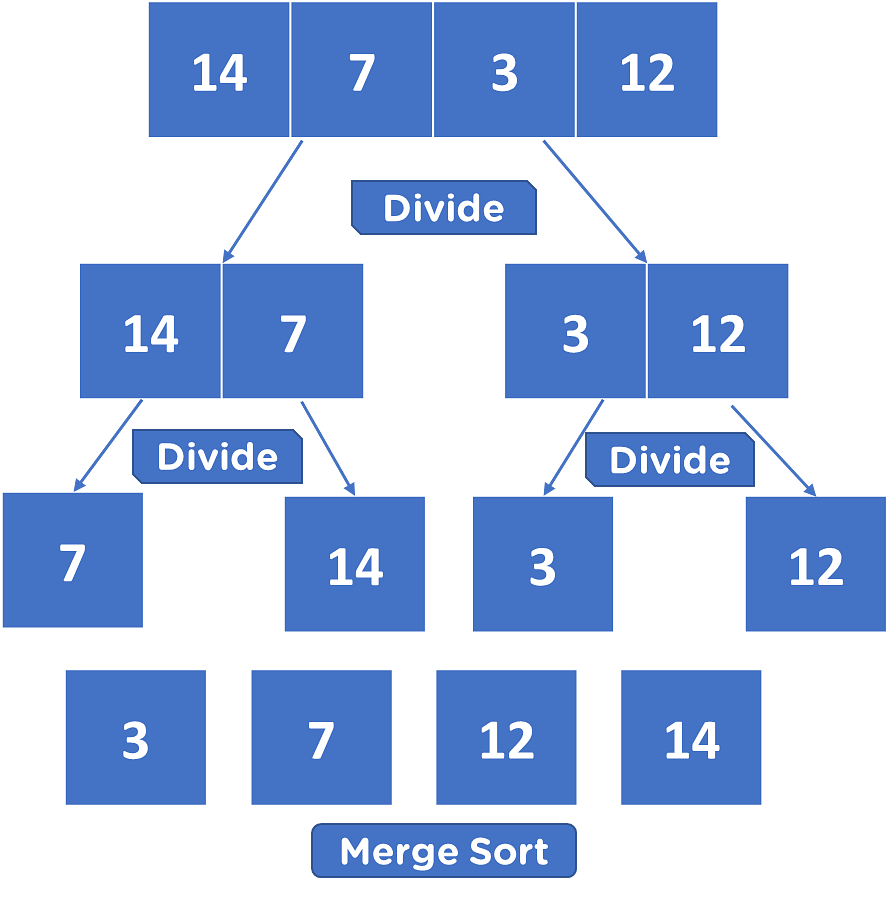
What is Merge Sort Algorithm How does it work, and More
In computer science, merge sort (also commonly spelled as mergesort) is an efficient, general-purpose, and comparison-based sorting algorithm.Most implementations produce a stable sort, which means that the relative order of equal elements is the same in the input and output.Merge sort is a divide-and-conquer algorithm that was invented by John von Neumann in 1945.
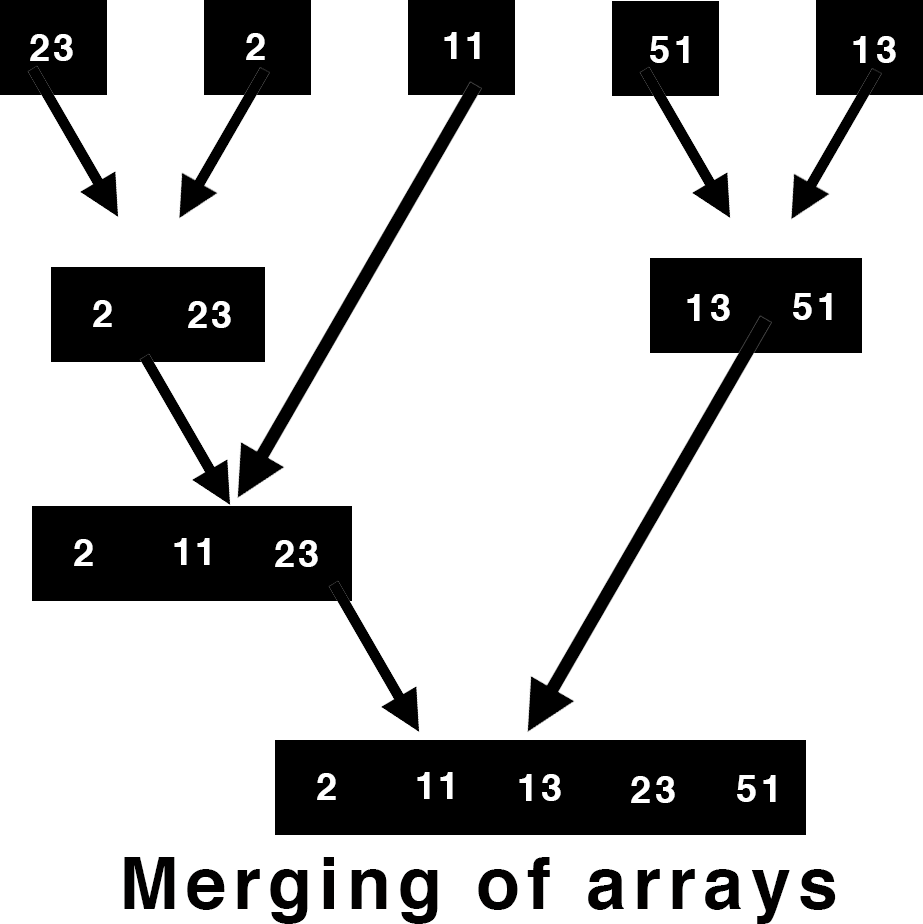
Merge Sort
Merge sort algorithm visualization. Implementation of merging algorithm Solution idea: Two pointers approach. After the conquer step, both left part A[l…mid] and right part A[mid + 1…r] will be sorted.Now we need to combine the solution of smaller sub-problems to build a solution to the larger problem, i.e., merging both sorted halves to create the larger sorted array.
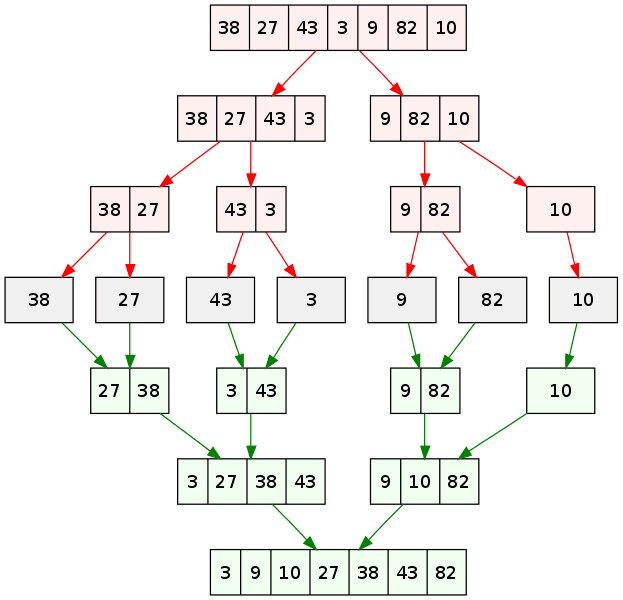
Penjelasan lengkap merge sort C++ Zona Pemrograman
Divide by finding the number q of the position midway between p and r .Do this step the same way we found the midpoint in binary search: add p and r , divide by 2, and round down.; Conquer by recursively sorting the subarrays in each of the two subproblems created by the divide step. That is, recursively sort the subarray array[p..q] and recursively sort the subarray array.
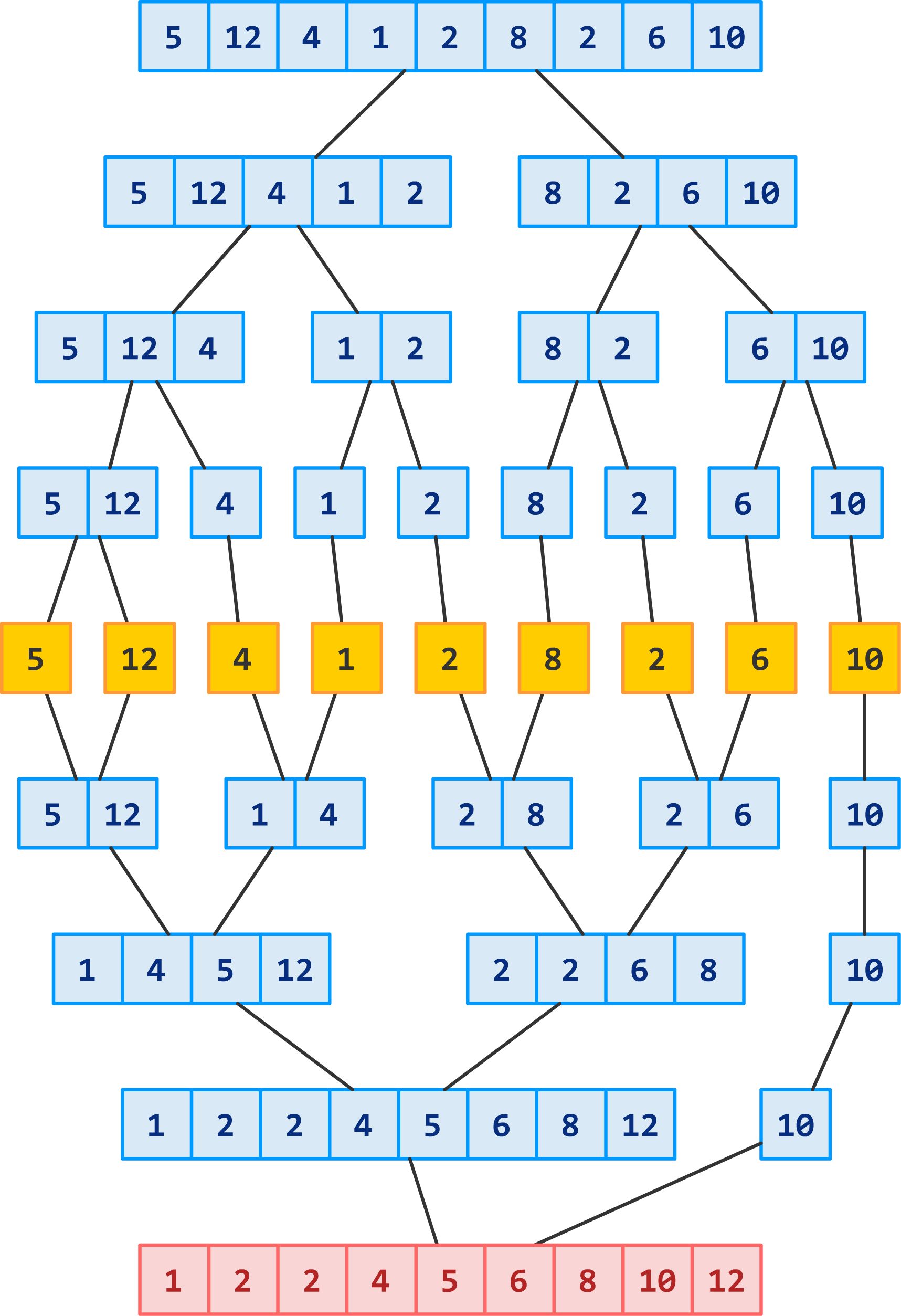
All About Mergesort
Merge Sort Algorithm. Merge sort keeps on dividing the list into equal halves until it can no more be divided. By definition, if it is only one element in the list, it is considered sorted. Then, merge sort combines the smaller sorted lists keeping the new list sorted too. Step 1: If it is only one element in the list, consider it already.
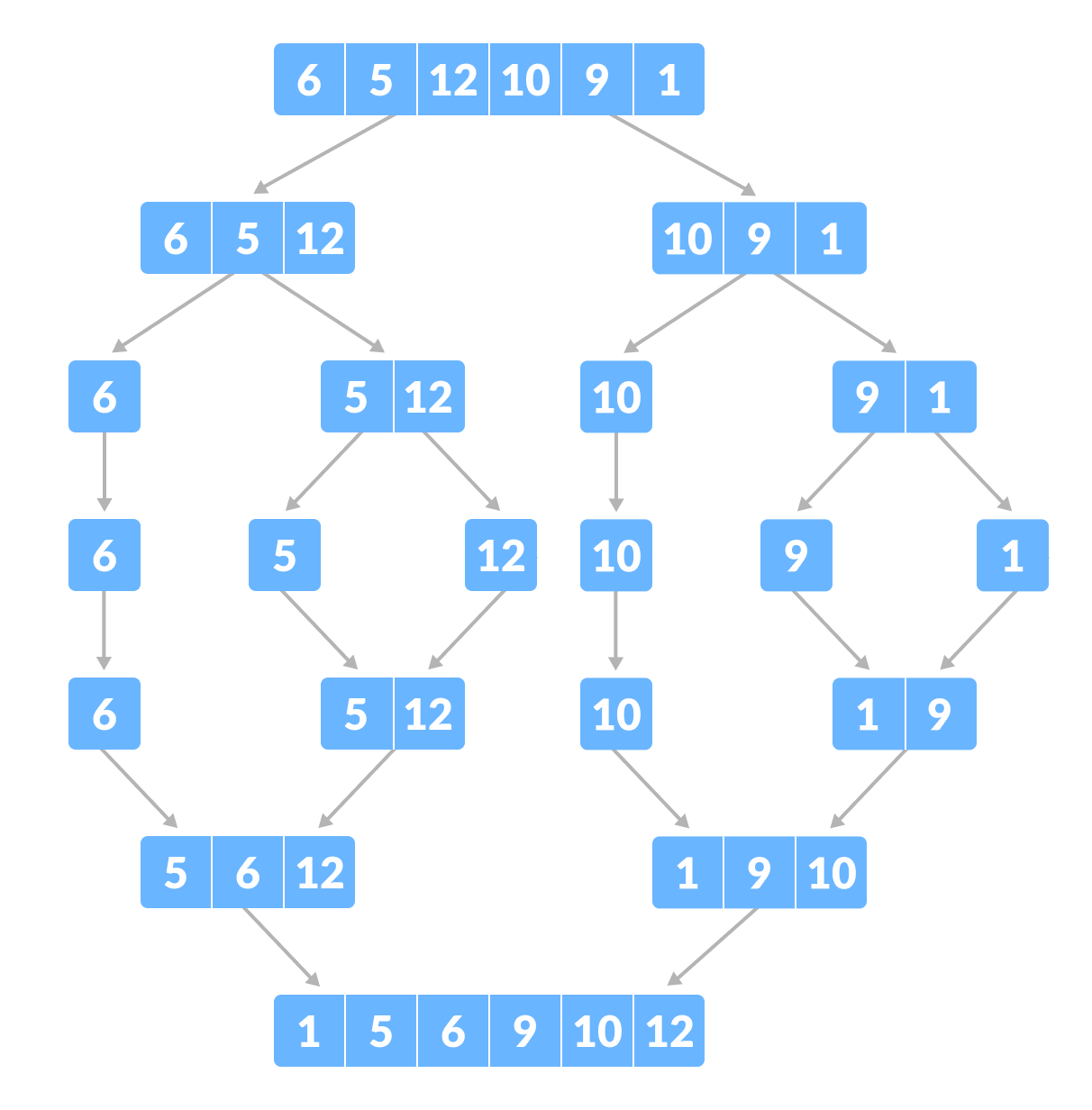
Merge Sort (With Code in Python/C++/Java/C)
hasil merge_sort cara pertama. Penjelasan Program. dari konsep diatas kita bisa memahami bahwa kita dapat mengurutkan list menggunakan metode (Algoritma) merge sort. kemudian kita membuat fungsi dengan parameter berupa list lalu fungsi tersebut mengembalikan inputan berupa list, kita menggunakan function annotation. disini kita bisa lihat kita mendefinisikan list kosong yang bernama sorted.

How to write Merge sort program in Java ? KK JavaTutorials
The time complexity of creating these temporary array for merge sort will be O (n lgn). Since, all n elements are copied l (lg n +1) times. Which makes the the total complexity: O (n lgn) + O (n lgn) = O (2n lgn). And we know that constants doesn't impact our complexity substantially.
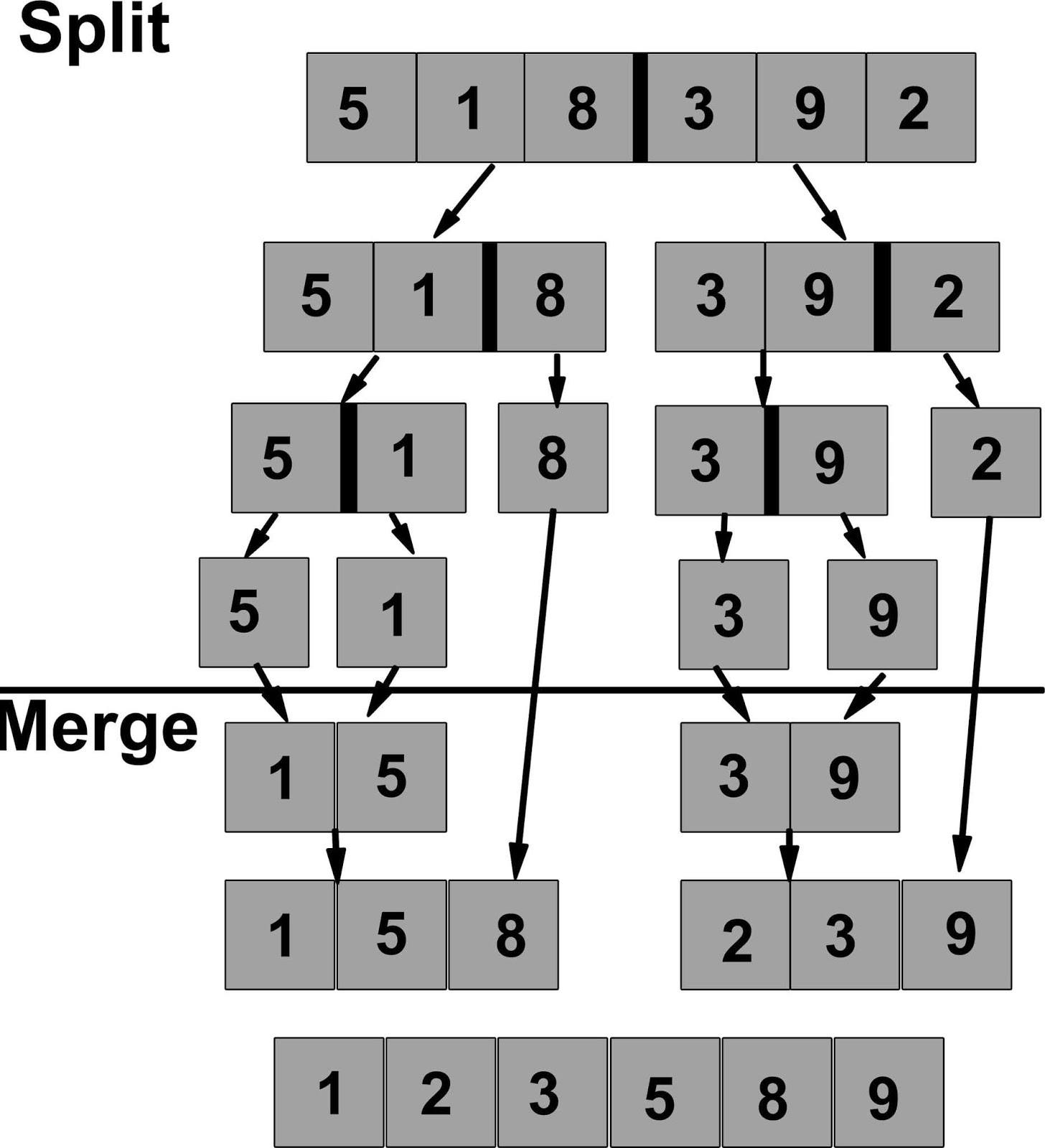
Penjelasan lengkap merge sort C++ Zona Pemrograman
Cara Kerja Algoritma Merge Sort. Berikut adalah langkah-langkah utama dalam algoritma Merge Sort: Pembagian (Divide): Langkah pertama dalam Merge Sort adalah membagi data yang akan diurutkan menjadi dua bagian seimbang. Algoritma ini terus membagi data menjadi bagian-bagian lebih kecil hingga setiap bagian hanya berisi satu elemen.
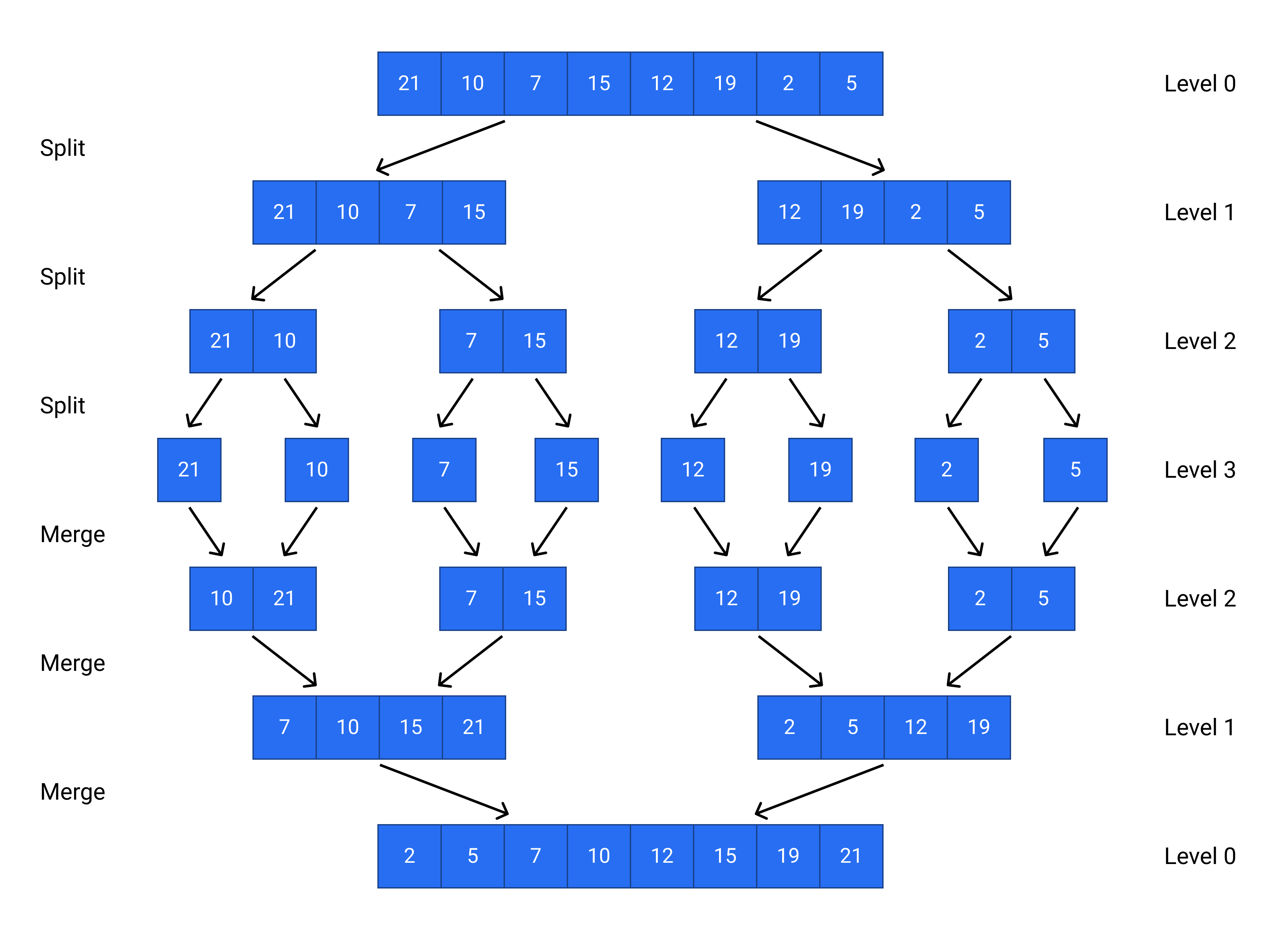
Sorting Algorithms (Quick Sort, Merge Sort) DSA Tutorials
Merge Sort Java Source Code. The following source code is the most basic implementation of Merge Sort. First, the method sort () calls the method mergeSort () and passes in the array and its start and end positions. mergeSort () checks if it was called for a subarray of length 1. If so, it returns a copy of this subarray.
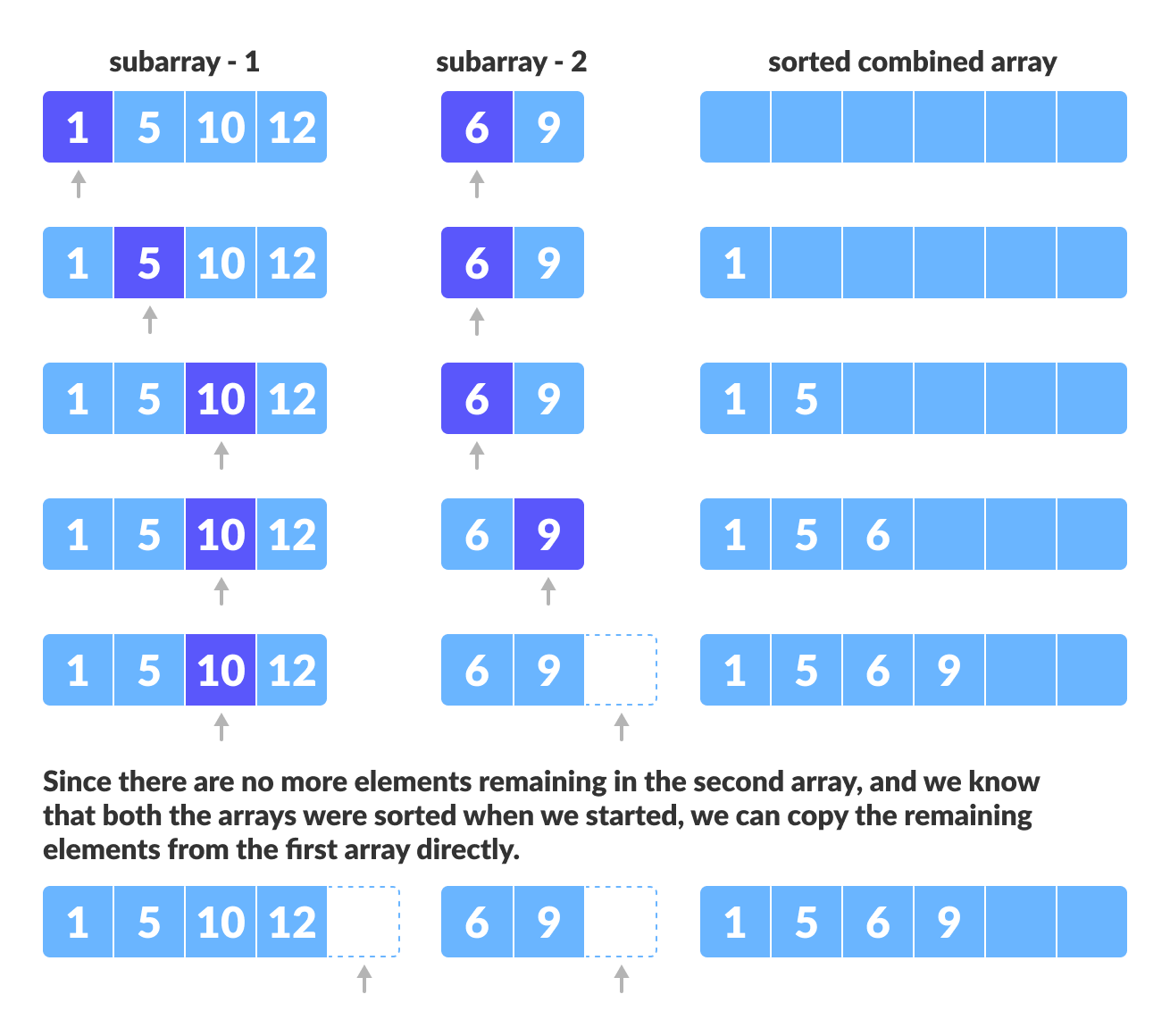
Merge Sort Algorithm
In the merge sort algorithm implementation, recursion occurs in the breaking down of lists. To ensure all partitions are broken down into their individual components, the merge_sort function is called, and a partitioned portion of the list is passed as a parameter. The merge_sort function returns a list composed of a sorted left and right.
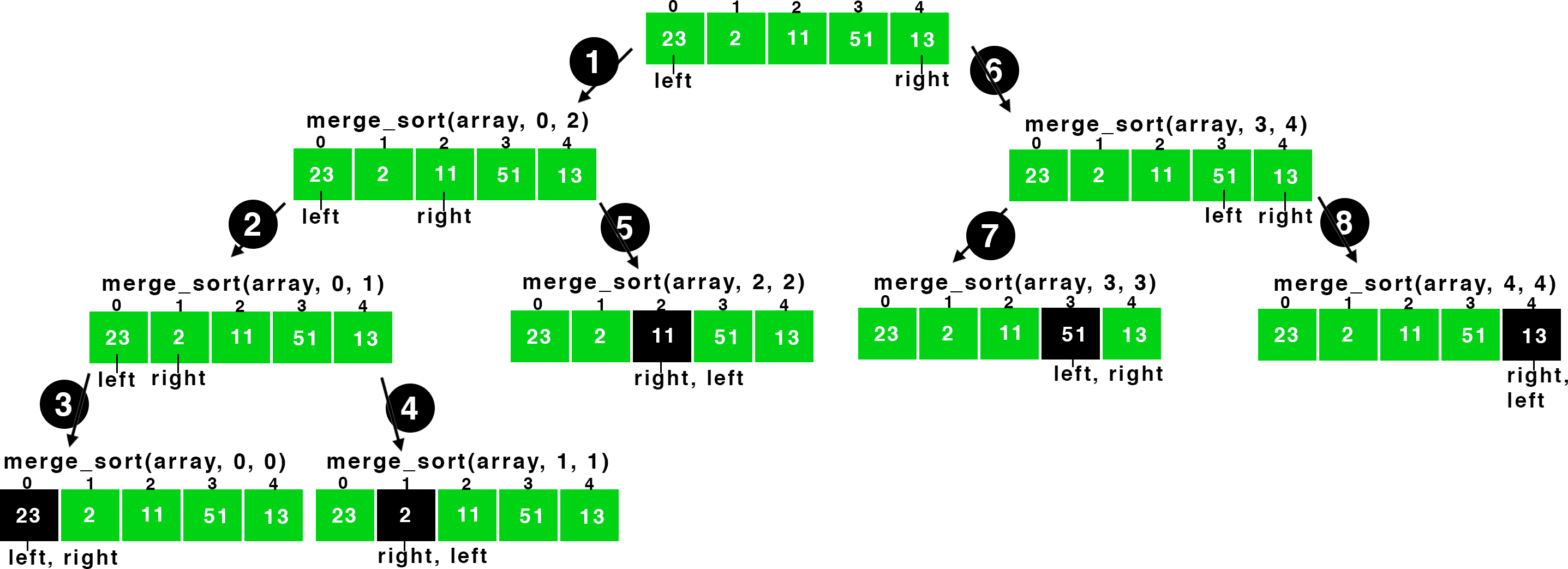
Merge Sort
Merge Sort is a divide-and-conquer algorithm. It divides the input array into two halves, calls itself the two halves, and then merges the two sorted halves. The merge() function is used for merging two halves. The merge(arr, l, m, r) is a key process that assumes that arr[l..m] and arr[m+1..r] are sorted and merges the two sorted sub-arrays into one.